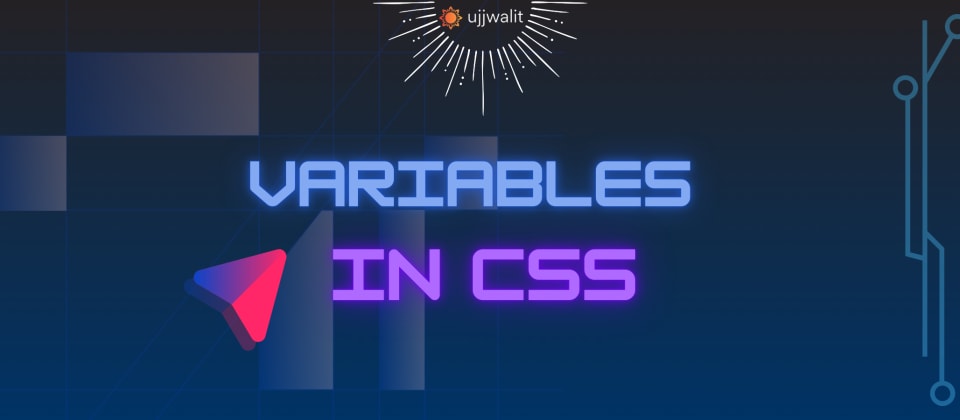
Variables in CSS
Hey Devs!
Making a website is never an easy task. You have to think of the design, the color palette, and the styling of various elements like text, images, buttons, and many more. Yet, what's more troubling is modifying it later. Imagine changing the color palette of a site from blue to turquoise. You would have to go through the CSS and update the color manually for every element that uses that shade of blue.
That’s not just time-consuming—it’s also risky. You might miss a few places, break consistency, or introduce bugs.
But thankfully, CSS follows the DRY principle—Don’t Repeat Yourself—and one of the most effective tools that help with this is CSS variables. In this blog, we’ll understand:
- What are variables?
- How to use variables?
- What is the
:root
selector? - How to define and organize variables efficiently
What Are Variables?
Variables in CSS (also called custom properties) let you store a value—like a color, font size, or margin—in one place and reuse it anywhere else in your stylesheets.
Instead of writing styles and properties repeatedly:
button {
background-color: #40E0D0;
}
a {
color: #40E0D0;
}
.card-header {
border-color: #40E0D0;
}
You define the color once:
--primary-color: #40E0D0;
And use it wherever needed:
button {
background-color: var(--primary-color);
}
a {
color: var(--primary-color);
}
.card-header {
border-color: var(--primary-color);
}
This not only keeps your CSS cleaner but also allows you to change your entire site’s theme by updating a few variables—rather than hundreds of style rules. It makes things easy to modify and organize.
How to Use Variables?
CSS variables are very easy to use. You just follow a two-step process:
- Define the variable considering its scope (Local and Global)
- Use the variable wherever needed
Here’s a basic example:
:root {
--primary-color: #40E0D0;
--secondary-color: #1f2937;
--font-stack: 'Poppins', sans-serif;
}
To use the variables:
body {
font-family: var(--font-stack);
background-color: var(--primary-color);
color: var(--secondary-color);
}
The var()
function is what lets you access the value of a variable. If you’re familiar with other programming languages, this is just like calling a variable’s value.
Note: Remember, CSS variables are case-sensitive. That means
--color
and--Color
are different variables.
What Is the :root
Selector?
The :root
pseudo-class refers to the top-level element of your HTML document. In most cases, this is equivalent to the <html>
tag.
So why do we define variables inside :root
?
Because it gives those variables global scope—which means they can be accessed from anywhere in your CSS.
Example:
:root {
--font-size-base: 16px;
--border-radius: 8px;
}
Now, whether you're styling a header, footer, button, or form input, you can use --font-size-base
or --border-radius
without redefining them.
It’s like creating a central theme or configuration file, but for your CSS.
Defining Variables: Global vs Local
To define a variable, you must remember three things:
- It must start with
--
(two hyphens). - A variable is case-sensitive in CSS. That means
--color
and--COLOR
are two separate variable names. - CSS variables can be global or local, depending on where you define them.
1. Global Variables
Global variables are declared inside the :root
selector. These are accessible from any part of your stylesheet.
:root {
--heading-color: #111;
--paragraph-color: #333;
}
Use case: When you want consistency across your entire site.
2. Local Variables
Local variables are defined within a specific selector. These are available only within that selector and its children.
.card {
--card-bg: #ffffff;
background-color: var(--card-bg);
}
This is useful when certain components need unique styling without affecting the global theme.
Why Use CSS Variables?
CSS variables aren't just a "nice to have"—they solve real problems in scaling and maintaining a website.
Here’s why you should consider using them:
- One place to change values: Update a color, spacing, or font just once.
- Consistent design system: Ensures your whole UI follows a unified theme.
- Easier maintenance: Avoids duplication and human error when editing.
- Supports theming: You can dynamically switch between light and dark themes by toggling variable values.
- Component-friendly: Combine with frameworks like Tailwind, or vanilla CSS, for scalable component-based systems.
Practical Tip: Organize Your Variables
As your project grows, your variable list might get long. Organizing them properly makes your CSS easier to navigate. Here’s a structure you can follow:
:root {
/* Color Palette */
--primary-color: #40E0D0;
--secondary-color: #1f2937;
--accent-color: #fbbf24;
/* Typography */
--font-heading: 'Poppins', sans-serif;
--font-body: 'Inter', sans-serif;
--font-size-base: 16px;
/* Layout */
--max-width: 1200px;
--border-radius: 8px;
--spacing-unit: 1rem;
}
You can even split them into separate files if you're using a preprocessor like SCSS or manage them using PostCSS in larger projects.
Conclusion
Learning to use CSS variables properly is a small investment with massive payoffs. You’ll not only write cleaner code but also build a system that’s easier to maintain and scale.
So the next time you start a project, don’t hard-code everything. Use variables. Create a theme. Make your CSS flexible.
And remember - design evolves, but a good system adapts.
Enjoy the content here?
Sign up on our platform or join our WhatsApp channel here to get more hands-on guides like this, delivered regularly.
See you in the next blog. Until then, keep practicing and happy learning!
6 Reactions
0 Bookmarks