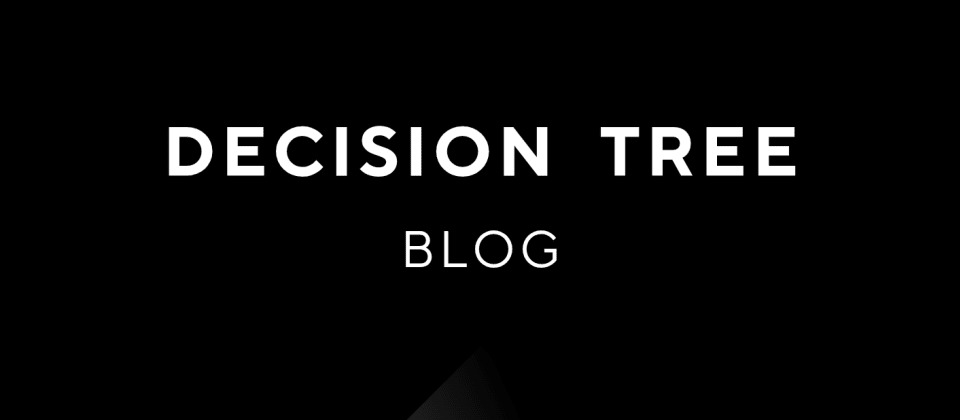
Understanding Decision Trees – A Simple Guide with Real-life Examples
Planned Structure of the Blog
Part 1: What is a Decision Tree?
Part 2: How Does a Decision Tree Work?
Part 3: Real-Life Example (Non-technical + Technical)
Part 4: Key Terminology (Root Node, Leaf Node, Splits, etc.)
Part 5: Advantages and Disadvantages
Part 6: Overfitting, Pruning & Tips
Part 7: Decision Tree in Machine Learning (with Scikit-learn/Pseudocode)
Part 8: Summary & Final Thoughts
Part 1: What is a Decision Tree?
A decision tree is a tool that helps you make decisions based on asking a series of yes/no or multiple-choice questions. It starts with one question and, depending on the answer, it leads to another question—until a final decision is reached.
Why is it called a "tree"?
Because it looks like an upside-down tree:
- The first question is like the root of the tree.
- Each answer branches out to more questions.
- The end result is like a leaf of the tree.
Real-Life Example (Basic):
Let’s say you’re deciding what to wear today:
Is it cold?
- Yes → Wear a jacket
- No → Next question
Is it raining?
- Yes → Take an umbrella
- No → Wear sunglasses
This is a decision tree you’re using in everyday life!
Part 2: How Does a Decision Tree Work?
Now that you know a decision tree is like a flowchart of questions and decisions, let’s dive a bit deeper into how it actually works, especially in the context of data and decision-making.
Step-by-Step Working of a Decision Tree:
Imagine you have data and you want to use it to make decisions. The decision tree helps by splitting the data based on the most useful questions. Here's how it works:
Step 1: Choose the Best Question (a.k.a. "Split")
The tree starts at the root, which is the first and most important question. This question splits the data into groups that are more "pure" — meaning the items in each group are more similar.
Let’s say you run a fruit shop and want to classify fruits based on their features (like color, size, and texture). The decision tree might start with:
Is the color red?
- Yes → Apples, Strawberries
- No → Bananas, Oranges
The tree chooses this question because it helps separate the fruits in the best way.
Step 2: Keep Splitting
Each group is then split again with another question:
Red fruits → Are they small?
- Yes → Strawberry
- No → Apple
You keep asking questions until you reach a point where there’s nothing more to split — either:
- All the data in that branch belongs to one class
- Or you’ve reached a certain limit (e.g., max depth)
How Does the Tree Know Which Question Is Best?
Good question! Behind the scenes, the tree uses math to decide which question is best at each step. It uses things like:
- Gini Impurity
- Entropy / Information Gain
- Gain Ratio
Don't worry about the formulas yet — just know that these are ways to measure how "pure" or useful a split is. The tree always picks the most helpful question first.
Visualizing It:
Here’s a very simple visual example of a decision tree to decide if someone will play tennis:
Is it sunny?
├── Yes: Is humidity high?
│ ├── Yes → No (Won’t play)
│ └── No → Yes (Will play)
└── No → Yes (Will play)
At every stage, the tree looks for the question that best divides the data so that each resulting group is more uniform — that means fewer mixed-up results.
Part 3: Real-Life Example of a Decision Tree (Non-technical + Technical)
Decision trees are so powerful because they work just like how humans make decisions: by asking questions. Let’s look at both a real-life example (non-technical) and a technical/data example so you get the full picture.
Non-Technical Example: Choosing a Weekend Activity
Let’s say you're deciding what to do on the weekend. Here's a simple decision tree for that:
Is the weather good?
├── Yes: Are you feeling energetic?
│ ├── Yes → Go hiking
│ └── No → Go for a picnic
└── No: Do you feel like being social?
├── Yes → Go to the movies
└── No → Stay home and read
Breakdown:
- Start with a simple question ("Is the weather good?")
- Depending on the answer, you go down different paths
- Each path ends with an action (your final decision)
This kind of logic can be represented as a decision tree — and machines can use the same structure to make predictions!
Technical Example: Predicting if a Student Will Pass an Exam
Now, let’s see a basic machine learning example.
Suppose you have a dataset of students, and you want to predict whether a student will pass or fail based on these features:
- Hours studied
- Attendance
- Completed assignments
Here’s a simplified version of what a decision tree might look like:
Has the student studied more than 4 hours?
├── Yes: Has attendance been above 75%?
│ ├── Yes → PASS
│ └── No → PASS (but maybe just barely)
└── No: Did they complete all assignments?
├── Yes → MAYBE PASS
└── No → FAIL
The tree has learned to ask the right questions based on patterns in the data. It follows a path until it reaches a decision — in this case: Pass, Maybe Pass, or Fail.
Key Takeaways:
- In both examples, the tree breaks the decision into smaller and simpler questions.
- Each question splits the problem into easier parts.
- At the end of each path is a final decision (called a leaf node in the tree).
Part 4: Key Terminology in Decision Trees (Explained Simply)
To fully understand how decision trees work — and to be able to talk about them clearly — it’s important to learn some basic terms used in decision trees. Don’t worry, these sound technical but are very easy to understand when you break them down.
1. Root Node
This is the first question or decision in the tree.
Think of it as the starting point.
📌 Example:
In the fruit example: “Is the color red?” — this is the root node.
2. Decision Node
A point in the tree where a decision is made, and it branches out into more questions or results.
📌 Example:
“Is the fruit small?” is a decision node that follows from “Is the color red?”
3. Leaf Node (Terminal Node)
This is where the tree ends, and a final decision or output is made.
📌 Example:
“Apple” or “Banana” are leaf nodes — they don’t lead to more questions.
4. Split
When a decision is made and the data is divided into two or more parts, it’s called a split.
📌 Example:
Splitting data into “Yes” and “No” groups based on the question “Is color red?”
5. Branch
Each possible answer to a question (node) creates a branch.
📌 Example:
From “Is it raining?”, you can have:
- Yes → Stay in
- No → Go out
These two are branches.
6. Gini Impurity / Entropy / Information Gain (Optional for general readers)
These are mathematical formulas used to decide which question to ask next (i.e., how to split). They measure:
- How mixed the data is (Gini or Entropy)
- How much information you gain by splitting (Information Gain)
📌 You don’t need to memorize the formulas — just know they help the tree find the best questions.
7. Depth of the Tree
This is the number of levels in the tree from root to leaf.
📌 Example:
If your tree has 3 questions before it reaches an answer, its depth is 3.
8. Subtree
A part of the tree that is itself a smaller tree. Every decision node can be thought of as the root of its own subtree.
📌 Helpful when discussing pruning or simplification (which we’ll cover soon).
Summary of Key Terms:
Term | Simple Meaning |
---|---|
Root Node | First question or decision |
Decision Node | A question that splits the data further |
Leaf Node | Final result or answer |
Split | A decision that divides data |
Branch | A path or direction from a decision |
Depth | Number of levels in the tree |
Subtree | A smaller tree inside the big tree |
Part 5: Advantages and Disadvantages of Decision Trees
Just like any tool or method, decision trees have their strengths and weaknesses. In this part, we’ll cover both so you understand when and why to use them — and when you might want to be careful.
Advantages of Decision Trees
-
Easy to Understand and Interpret
Even non-technical people can understand a decision tree. It follows a logical, question-based structure that mirrors human thinking.📌 Example:
"Is it raining?" → If yes, take an umbrella. This makes intuitive sense! -
No Need for Feature Scaling or Normalization
Some machine learning models need data to be normalized (e.g., between 0 and 1). Decision trees don’t. They can handle raw numbers and categories as they are. -
Works for Both Classification and Regression
You can use a decision tree to classify (e.g., pass/fail, yes/no, dog/cat).
You can also use it to predict numbers (like sales or price). -
Handles Both Numerical and Categorical Data
Decision trees can easily work with data that includes:- Numbers (age, income)
- Categories (color, type, brand)
Disadvantages of Decision Trees
-
Prone to Overfitting
If a tree grows too deep, it may memorize the training data instead of learning patterns. This makes it perform poorly on new data.📌 Overfitting means: “Great in practice, terrible in real life.”
-
Unstable (Sensitive to Small Changes)
A small change in the data can cause a completely different tree structure. This makes decision trees less stable than other methods. -
Can Be Biased with Imbalanced Data
If some classes are much more frequent than others, the tree might get biased toward them.📌 Example:
If 90% of emails are not spam, the tree might just always say “not spam.” -
Not Always the Most Accurate
Although easy to understand, decision trees often don’t perform as well as more advanced models like Random Forests, Gradient Boosting, or Neural Networks, especially on complex datasets.
Part 6: Overfitting, Pruning & Tips to Improve Decision Trees
One of the biggest challenges with decision trees is that they can become too good at learning the training data — this is called overfitting. In this part, we’ll explain what overfitting is, how to prevent it using pruning, and share some useful tips to improve your decision tree models.
What is Overfitting?
Overfitting happens when your decision tree becomes too detailed and tries to learn every small detail and noise in the training data — even patterns that don’t matter.
📌 Example:
Imagine you're teaching a child to recognize dogs. If you show them 1,000 photos and they memorize every single one, they might not recognize a dog in a new photo that looks a bit different. That’s overfitting.
How to Fix Overfitting?
The main solution is pruning the tree.
What is Pruning?
Pruning means cutting down the size of the tree by removing branches that don’t help much. The goal is to make the tree simpler and more general, so it performs better on new data.
There are two types:
1. Pre-Pruning (a.k.a. Early Stopping)
Stop growing the tree early by setting rules like:
- Max depth of the tree (e.g., no more than 4 levels)
- Minimum samples required to split a node
- Minimum number of samples per leaf
📌 Example:
"Don’t split a node if it has fewer than 5 samples."
2. Post-Pruning (a.k.a. Reduced Error Pruning)
First grow the full tree, then go back and cut off parts of the tree that are not helping (based on validation data or accuracy tests).
📌 Example:
"If removing this branch improves accuracy or makes no difference, cut it off."
Tips to Improve a Decision Tree
Here are some practical ways to make your decision tree better:
-
Limit Tree Depth
Set a maximum depth so the tree doesn’t grow too long and complex. -
Use Cross-Validation
Test the model on multiple subsets of your data to make sure it works well generally, not just on one portion. -
Balance Your Data
If one class (e.g., "pass") appears way more often than another (e.g., "fail"), the tree might just always predict the common one. Use techniques like:- Resampling (oversampling/undersampling)
- Class weights
-
Try Ensemble Methods
If a single tree isn’t doing well, use many trees together! For example:- Random Forest: Builds many decision trees and takes the average or majority vote.
- Gradient Boosting: Builds trees one by one, each trying to fix the errors of the last one.
Part 7: Decision Trees in Machine Learning (with Simple Code Example)
Now that you understand the concepts, let’s see how decision trees are actually used in machine learning. This part will cover:
- How machines "learn" decision trees from data
- A simple real-world ML example
- A beginner-friendly Python code using Scikit-learn
How Machines Build a Decision Tree
When we use decision trees in machine learning, we don't manually write the questions. Instead, the algorithm automatically learns the best questions from the data.
Basic process:
- Feed labeled data (features + correct answers) to the algorithm.
- It figures out which questions split the data best at each step.
- It builds the tree from top to bottom until it's confident in its predictions.
Hours Studied | Attendance (%) | Pass/Fail |
---|---|---|
2 | 60 | Fail |
5 | 80 | Pass |
3 | 70 | Fail |
6 | 90 | Pass |
The decision tree algorithm will analyze the patterns and figure out that:
If "Hours Studied > 4", then most likely → Pass
Let’s See It in Python (Scikit-learn)
Here’s a simple and beginner-friendly example using Python's Scikit-learn library.
from sklearn.tree import DecisionTreeClassifier
from sklearn import tree
import matplotlib.pyplot as plt
# Sample data
# Features: [hours_studied, attendance]
X = [
[2, 60],
[5, 80],
[3, 70],
[6, 90],
[1, 50],
[7, 95]
]
# Labels: 0 = Fail, 1 = Pass
y = [0, 1, 0, 1, 0, 1]
# Create and train the decision tree
model = DecisionTreeClassifier()
model.fit(X, y)
# Predict a new student's result
print(model.predict([[4, 75]])) # Example: Might predict [1] for Pass
# Visualize the decision tree
plt.figure(figsize=(10, 6))
tree.plot_tree(model, feature_names=["Hours Studied", "Attendance"], class_names=["Fail", "Pass"], filled=True)
plt.show()
Explanation:
- X: Input features (hours studied and attendance).
- y: Labels (pass/fail).
- fit(): Trains the decision tree on the data.
- predict(): Makes a prediction for a new student.
- plot_tree(): Shows the tree visually.
Real-World Applications:
- Predicting loan approval
- Diagnosing medical conditions
- Deciding if a customer will churn
- Classifying emails as spam or not
- Recommending products or services
Common Libraries That Use Decision Trees:
- Scikit-learn (Python)
- XGBoost / LightGBM / CatBoost (for gradient boosting trees)
- Weka (Java)
- R’s rpart and party packages
Part 8: Summary & Final Thoughts on Decision Trees
We’ve covered a lot about decision trees — from basic concepts to real-world use cases. This final part brings everything together so you walk away with a clear and complete picture.
Quick Recap
Here’s what you learned throughout this guide:
Part 1: Introduction
A decision tree is like a flowchart that helps you make decisions or predictions by asking questions.
Part 2: How It Works
- It splits data based on the best features/questions.
- It continues asking questions until a decision (leaf node) is reached.
Part 3: Real-Life Examples
From daily life choices (e.g., weekend plans) to student performance prediction, decision trees mirror human reasoning.
Part 4: Key Terminology
Terms like root node, leaf node, split, branch, and depth help describe parts of a tree.
Part 5: Pros and Cons
Easy to understand and use.
Can overfit and be unstable with noisy or complex data.
Part 6: Overfitting & Pruning
- Prevent overfitting with pre-pruning (limit tree size) or post-pruning (remove unnecessary branches).
- Tips: Use ensembles, balance data, limit depth.
Part 7: ML Implementation
- You can build decision trees in Python with libraries like Scikit-learn.
- They can classify or predict based on data inputs.
Part 8: Real-World Applications
- Used in banking, healthcare, e-commerce, education, and more.
- Also a base for more powerful models like Random Forests and Gradient Boosting.
💬 Final Thoughts
Decision trees are one of the most intuitive and versatile tools in machine learning. They’re great for:
- Beginners who want to learn how models work
- Businesses that need interpretable AI
- Engineers who want fast and scalable solutions
But remember: for bigger and more complex datasets, you’ll often need pruning or ensemble methods to get the best results.
🛠 Bonus Tip:
If you're going deeper into data science, try exploring:
Random Forest: Combines many decision trees
XGBoost: Great for competitions and performance
Hyperparameter tuning: Makes your trees smarter
Thanks for reading.......Stay Tuned
5 Reactions
2 Bookmarks