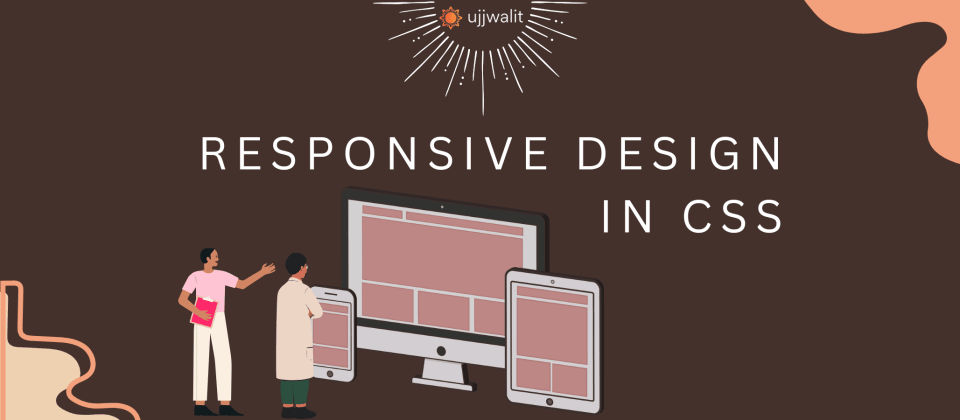
Responsive Design In CSS
Hey Devs!
What makes a website good?
Is it the content? The information it holds? Its colors? Its images?
All of those are important -no doubt. But what really makes a website great is how well it works for everyone, everywhere, on every device. If your site looks amazing on a laptop but totally breaks on a phone, you've lost half your audience already. That's where responsive design steps in.
And the secret weapon for responsive design? Media queries.
What Are Media Queries?
Media queries are like smart filters in CSS. They allow your site to adapt by applying styles only when specific conditions are met -like screen width, height, orientation, user preferences (like dark mode), and more.
Think of media queries as a way to say:
"Hey browser, if the screen is small, do this. If the screen is wide, do that."
Here's the syntax:
@media (condition) {
/* styles go here */
}
Let's go over some of the most common and useful conditions you can use.
1. min-width and max-width
These are the most common media features. Use them to target screens of different sizes.
/* Tablet and up */
@media (min-width: 768px) {
body {
font-size: 1.2rem;
}
}
/* Mobile only */
@media (max-width: 600px) {
.navbar {
flex-direction: column;
}
}
Pro Tip: Use min-width
for mobile-first design. Start with mobile styles, and add enhancements as screen size grows.
2. Orientation
You can style your site differently based on whether the device is in portrait or landscape mode.
@media (orientation: landscape) {
.video-player {
width: 70vw;
}
}
This is super handy for tablets or mobile devices when people rotate their screens.
3. prefers-color-scheme
Want to support dark mode? Use this! Modern browsers let you detect the user's OS preference.
@media (prefers-color-scheme: dark) {
body {
background-color: #111;
color: #eee;
}
}
And for light mode:
@media (prefers-color-scheme: light) {
body {
background-color: white;
color: black;
}
}
4. resolution / pixel-ratio
You can target high-DPI screens (like Retina displays) to show higher-quality images.
@media (min-resolution: 192dpi) {
.logo {
background-image: url('logo-highres.png');
}
}
Or:
@media (-webkit-min-device-pixel-ratio: 2) {
.icon {
background-image: url('icon@2x.png');
}
}
Advanced: Combine Conditions
You can chain conditions using and
, not
, or commas (,
= or).
@media (min-width: 600px) and (orientation: portrait) {
.sidebar {
display: none;
}
}
@media (max-width: 500px), (orientation: landscape) {
body {
font-size: 0.9rem;
}
}
Additional Responsive Design Techniques
Media queries are crucial, but they're only part of the responsive design toolbox. Let's explore some additional techniques that help your site shine across devices.
1. Use Relative Units
Avoid fixed sizes like px
for fonts, paddings, and widths. Prefer:
em
/rem
for font sizes%
for widthsvw
/vh
for full viewport sizes
.container {
width: 80%;
padding: 2rem;
font-size: 1rem;
}
You can refer to this quick table to learn more about the units in CSS.
Unit | Type | Use Case Example | Best For |
---|---|---|---|
px | Absolute | padding: 20px | Precise control (not responsive) |
% | Relative | width: 80% of the parent element | Fluid layouts |
em | Relative | font-size: 1.2em (relative to parent element’s font-size) | Typography, padding/margin |
rem | Relative | font-size: 1.2rem (relative to root font-size) | Consistent scalable sizing |
vw | Viewport | width: 50vw (50% of viewport width) | Full-width layouts, spacing |
vh | Viewport | height: 100vh (100% of viewport height) | Hero sections, full-screen blocks |
vmin/vmax | Viewport | font-size: 3vmin (smallest viewport dimension) | Responsive typography/images |
fr | Grid-specific | grid-template-columns: 1fr 2fr | CSS Grid for fluid column sizing |
auto | Keyword | margin: auto | Centering, dynamic sizing |
ch | Relative | width: 60ch (based on character width) | Readable text blocks |
ex | Relative | height: 2ex (based on x-height of current font) | Rare; fine typography tweaks |
2. Flexbox & Grid Layouts
These layout systems are inherently responsive and adapt beautifully to different screen sizes.
Flexbox example:
.card-container {
display: flex;
flex-wrap: wrap;
gap: 1rem;
}
To learn more about CSS Flexbox refer here.
Grid example:
.grid-layout {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(250px, 1fr));
gap: 1rem;
}
To learn more about CSS Grid refer here.
3. Images that Scale
Make sure your images don't overflow or break your layout.
img {
max-width: 100%;
height: auto;
}
4. Responsive Typography
Use clamp()
to scale fonts based on screen width.
h1 {
font-size: clamp(1.5rem, 4vw, 3rem);
}
To learn more about CSS functions like clamp()
check out this blog here.
Project: Responsive Navbar
Now that we know the theory, let's build a practical example: a responsive navbar that adapts across screen sizes using nothing but CSS.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Hello, World!</title>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<nav class="navbar">
<h1 class="logo">Ujjwalit</h1>
<div class="nav-links">
<a href="#">Home</a>
<a href="#">About</a>
<a href="#">Contact</a>
</div>
</nav>
</body>
</html>
CSS (Base)
body {
padding: 25px;
font-family: Arial;
}
.navbar {
display: flex;
justify-content: space-between;
align-items: center;
padding: 16px 24px;
background-color: #333;
color: white;
}
.nav-links {
display: flex;
gap: 16px;
}
.nav-links a {
color: white;
padding: 10px;
border: 1px solid;
text-decoration: none;
font-weight: 500;
transition: 0.5s ease-in;
}
.nav-links a:hover {
background: white;
color: black;
}
CSS (Responsive)
@media (max-width: 600px) {
.navbar {
flex-direction: column;
align-items: flex-start;
gap: 12px;
}
.nav-links {
flex-direction: column;
width: 100%;
gap: 8px;
}
.nav-links a {
width: 100%;
text-align: center;
}
}
Try resizing your browser -the navbar morphs into a stacked mobile version. All done with CSS. No JavaScript needed.
Conclusion
Responsive design is about inclusion. You're not just designing for yourself - you're designing for everyone: phone users, tablet users, people in dark mode, those with limited vision, and more.
Media queries are powerful. Learn them. Use them. Love them.
They'll help you:
- Build websites that look great everywhere
- Adapt to user preferences
- Future-proof your design
Next time you're building a UI, don't just think about how it looks on your screen. Think about your friend's phone, or that random smart fridge in someone's kitchen.
Enjoy the content here?
Sign up on our platform or join our WhatsApp channel here to get more hands-on guides like this, delivered regularly.
See you in the next blog. Until then, keep practicing and happy learning!
5 Reactions
2 Bookmarks