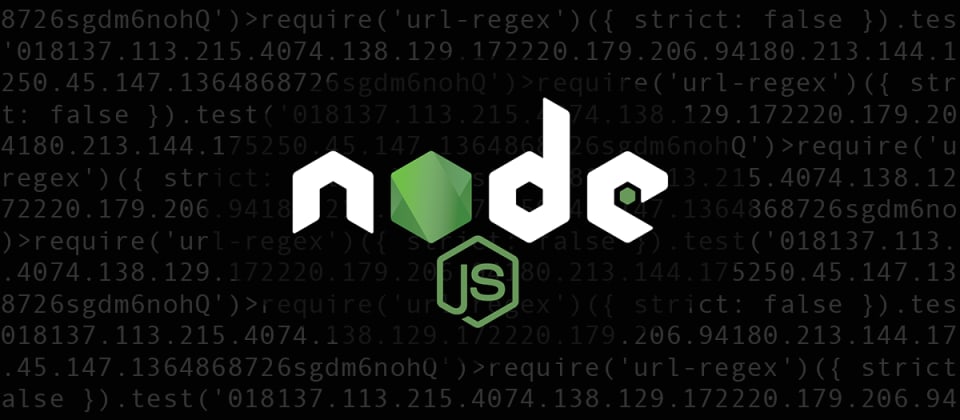
A Complete Guide to HTTP Methods and the URL Module in Node.js (with Examples)
Understanding HTTP Methods and the URL Module in Node.js
In modern web development, especially with Node.js, two concepts you'll work with often are HTTP methods and the URL module. These are foundational when building any web server, REST API, or even handling custom routing logic.
1. Introduction
-
What is HTTP?
HTTP (HyperText Transfer Protocol) is the foundation of communication on the web, allowing clients (like browsers) to request resources from servers. -
What is a URL?
A URL (Uniform Resource Locator) is an address that specifies the location of a resource on the web. -
Why understanding both is essential in web development?
Understanding HTTP and URLs is crucial for handling requests and responses effectively, ensuring seamless interaction between clients and servers. -
Quick Overview of Node.js
Node.js is a runtime environment that allows developers to run JavaScript on the server side. It is lightweight, scalable, and widely used for building web applications.
2. Understanding HTTP (HyperText Transfer Protocol)
HTTP Methods:
GET
– Retrieve data from the server.POST
– Send new data to the server.PUT
– Update existing data on the server.DELETE
– Remove data from the server.
HTTP Status Codes:
200 OK
– Request was successful.404 Not Found
– Resource was not found.500 Internal Server Error
– Server encountered an error.
Request vs Response:
- Request – Sent by the client; includes method, headers, and optional data.
- Response – Sent by the server; includes status code, headers, and data.
3. What is a URL?
Breakdown of a URL:
https://www.example.com:443/path?query=string#fragment
- Scheme (
https://
) – Protocol used for communication. - Host (
www.example.com
) – The domain name or IP address. - Port (
443
) – Specifies the communication channel (default for HTTPS). - Path (
/path
) – The location of a resource on the server. - Query (
?query=string
) – Parameters passed to the server. - Fragment (
#fragment
) – Internal page reference.
4. Setting Up Node.js
Installation Guide:
Install Node.js and npm:
Download and install from Node.js official site.
Verify Installation:
bash
node -v
npm -v
This guide will walk you through both topics in plain English, using only Node.js core modules (http
and url
), and include a step-by-step mini project that you can run and test locally.
💡 What Are HTTP Methods?
HTTP methods tell a server what action the client wants to perform on a resource (like a product, user, file, etc.).
🔍 Most Common Methods
Method | Purpose | Real-life Analogy |
---|---|---|
GET | Fetch or read data | Reading a blog post |
POST | Send data to be stored/created | Submitting a form or uploading a photo |
PUT | Replace or update existing data | Replacing a whole file with a new one |
DELETE | Remove existing data | Deleting an email |
🛠 Using HTTP Methods in Node.js
Node.js provides the http
module to create a server. Here’s a breakdown of how you receive and differentiate between methods:
const http = require('http');
const server = http.createServer((req, res) => {
const method = req.method; // GET, POST, etc.
const url = req.url; // Path and query string
console.log(`Received ${method} request on ${url}`);
res.end('Response from server');
});
server.listen(3000, () => {
console.log('Server running on http://localhost:3000');
});
Understanding the URL Module in Node.js
In this example:
req.method
tells you what the client is trying to do.req.url
tells you which path they're accessing.
🔗 Understanding the URL Module
The url
module helps parse and manipulate the request URL. This is especially useful for extracting:
- Pathnames (like
/products/123
) - Query parameters (like
?category=books
)
✅ Basic Example:
const url = require('url');
const address = 'http://localhost:3000/products?category=books&sort=price';
const parsedUrl = url.parse(address, true);
console.log(parsedUrl.pathname); // /products
console.log(parsedUrl.query); // { category: 'books', sort: 'price' }
🔐 Mini Project: "Secure Note Sharing API"
🧩 What It Does
- A user can POST a secret message (note) to the server.
- The server stores it temporarily in memory.
- A short URL (GET endpoint) is returned for retrieving the message once.
- After retrieval, the message is deleted (single-use).
- Demonstrates:
- HTTPS-style API usage
- Routing
- Non-blocking I/O
- Node.js architecture
📚 Concepts Covered
Concept | How It's Used |
---|---|
HTTP Methods | POST to store, GET to retrieve |
URLs | Custom route like /note/:id |
Node.js Architecture | Event-driven, non-blocking I/O with in-memory store |
Blocking vs Non-Blocking | Simulated using dummy file read/write or CPU delay |
🛠️ Tech Stack
- Node.js (vanilla + Express)
- nanoid for generating unique IDs
- Simulated blocking/non-blocking tasks for concept demonstration
🗂 Project Structure
secure-note-api/
├── index.js
├── package.json
📦 Step-by-Step Instructions
- Setup
mkdir secure-note-api
cd secure-note-api
npm init -y
npm install express nanoid
- Code
const express = require('express');
const fs = require('fs');
const { nanoid } = require('nanoid');
const app = express();
const PORT = 3000;
app.use(express.json());
const noteStore = {}; // In-memory storage
// Blocking function (simulated)
function blockingTask() {
const start = Date.now();
while (Date.now() - start < 200) {} // Blocks for 200ms
}
// Non-blocking version
function nonBlockingTask(callback) {
setTimeout(callback, 200); // Doesn't block the event loop
}
// POST /note - Create a note (Blocking simulation)
app.post('/note', (req, res) => {
blockingTask(); // simulate CPU-intensive work
const { message } = req.body;
if (!message) return res.status(400).json({ error: 'Message required' });
const id = nanoid(6);
noteStore[id] = message;
res.status(201).json({
noteUrl: `http://localhost:${PORT}/note/${id}`,
});
});
// GET /note/:id - Retrieve a note (Non-blocking simulation)
app.get('/note/:id', (req, res) => {
const id = req.params.id;
nonBlockingTask(() => {
const message = noteStore[id];
if (!message) {
return res.status(404).json({ error: 'Note not found or already viewed' });
}
delete noteStore[id]; // One-time read
res.status(200).json({ message });
});
});
// Server start
app.listen(PORT, () => {
console.log(`Secure Note API running at http://localhost:${PORT}`);
});
📦 Mini Project Summary: Secure Note Sharing API
This project is a mini Node.js API designed to demonstrate key backend concepts, including HTTP methods, URL routing, Node.js architecture, and blocking vs non-blocking operations.
🔧 Functionality
POST /note
- Accepts a JSON body containing a
"message"
. - Simulates a blocking task (CPU-bound) before storing the message.
- Returns a unique short URL (like
/note/abc123
) that can be used to retrieve the message.
GET /note/:id
- Simulates a non-blocking task (via
setTimeout
). - Retrieves and deletes the note (one-time read).
- If the note doesn't exist (already viewed or invalid ID), returns 404 Not Found.
🔍 Key Concepts Demonstrated
Concept | Implementation |
---|---|
HTTP Methods | POST to create, GET to retrieve |
Custom URL Routing | GET /note/:id dynamically handles unique IDs |
Blocking I/O | Simulated with a busy-wait loop in blockingTask() |
Non-Blocking I/O | Simulated with setTimeout() in nonBlockingTask() |
Node.js Architecture | Event-driven, async handling via callback patterns |
In-Memory Storage | Simple JavaScript object used as temporary store |
💡 Real-World Applications
This kind of architecture is useful in:
- 🔐 Secret/temporary message sharing
- ⬇️ One-time download links
- 🔑 Single-use API keys
- 📚 Teaching how Node.js handles asynchronous behavior
``
Thanks for reading ~ Jai hanuman
5 Reactions
1 Bookmarks