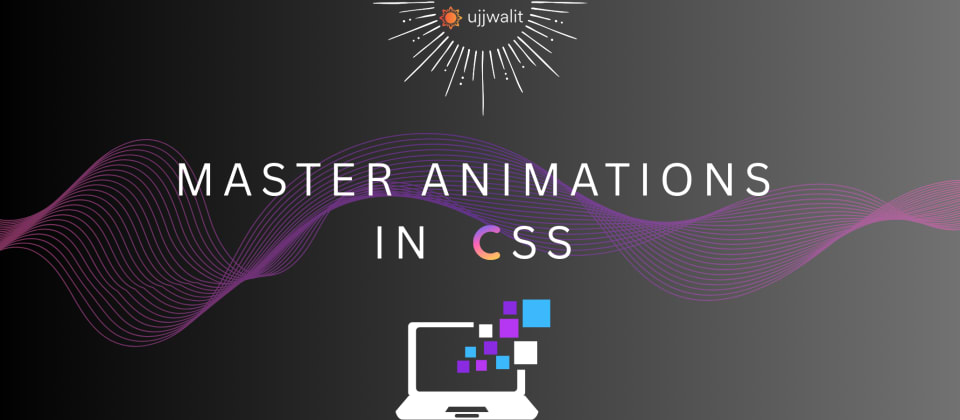
Master Animations In CSS
Hey Devs!
You know how sometimes a new feature you build just doesn't grab the attention it deserves? Transitions are neat for visual cues, but they need a trigger and have their limits. That's where CSS animations step in, giving your website a life of its own, with elements that seem to have a natural movement.
Today, we're diving into the world of CSS animations and learning how to create simple yet engaging effects, including a quick loading screen project.
Here's what we'll cover:
@keyframes
- timing functions
- animation vs transitions
- animation shorthand
- controlling animation playback
- practical effects and use cases
- project
What are Animations?
In CSS, an animation is a technique to smoothly change the styles of an HTML element over a set period. These changes can involve anything visual, like where an element is, its size, color, or how transparent it is.
A CSS animation has two main parts:
-
@keyframes
rule: This is where you define the different stages of the animation, specifying the styles at various points in time (usually as percentages). Think of these as the key moments of your animation. -
Animation properties: These are applied to the HTML element to tell it how to run the animation. The important ones include:
animation-name
: Links to the name you gave your@keyframes
rule.animation-duration
: How long the entire animation cycle takes.animation-timing-function
: Controls the speed curve of the animation (e.g., constant speed, starts slow then fast).animation-delay
: How long to wait before the animation starts.animation-iteration-count
: How many times the animation should repeat (once, twice, infinite, etc.).animation-direction
: Whether the animation plays forwards, backwards, or alternates.animation-fill-mode
: Determines how the element looks before and after the animation runs.
CSS animations are a great way to make your website feel more interactive and provide smooth visual feedback to users.
1. Defining Animation Steps: The @keyframes
Rule
The @keyframes
rule is where you lay out the blueprint for your animation. You give your animation a name, and then you define the CSS styles that the element should have at different points during the animation's timeline.
Example 1: fadeIn
This @keyframes
rule creates a simple fade-in effect.
/* Define the steps for a fade-in effect */
@keyframes fadeIn {
from { /* 'from' is the start (0% mark) */
opacity: 0; /* Element starts fully transparent */
}
to { /* 'to' is the end (100% mark) */
opacity: 1; /* Element ends fully opaque */
}
}
@keyframes fadeIn { ... }
: This line declares an animation and names itfadeIn
. You'll use this name later to apply the animation to an element.from { ... }
: These styles define what the element looks like at the very beginning (0%) of the animation.opacity: 0;
: Sets the element to be completely transparent. Theopacity
property ranges from0
(invisible) to1
(fully visible).
to { ... }
: These styles define what the element looks like at the very end (100%) of the animation.opacity: 1;
: Sets the element to be fully visible.
- Result: When you apply the
fadeIn
animation to an element, it will gradually become visible, going from fully transparent to fully opaque.
Example 2: moveAndColor
This rule shows a more complex animation that involves changing the background color and moving the element horizontally.
/* Define steps for movement and color change */
@keyframes moveAndColor {
0% { /* Start point */
background-color: blue;
transform: translateX(0); /* Start at original horizontal position */
}
50% { /* Mid-point (halfway through duration) */
background-color: yellow;
transform: translateX(100px); /* Move 100px to the right */
}
100% { /* End point */
background-color: red;
transform: translateX(0); /* Return to original horizontal position */
}
}
@keyframes moveAndColor { ... }
: Declares an animation namedmoveAndColor
.0% { ... }
: These are the styles at the beginning of the animation.background-color: blue;
: Sets the initial background color.transform: translateX(0);
: Thetransform
property lets you modify the appearance of an element.translateX()
specifically moves it along the horizontal axis.0
means no movement from its original position.
50% { ... }
: These are the styles halfway through the animation.background-color: yellow;
: Changes the background color at the midpoint.transform: translateX(100px);
: Moves the element 100 pixels to the right.
100% { ... }
: These are the styles at the end of the animation.background-color: red;
: Changes the background color again.transform: translateX(0);
: Moves the element back to its original horizontal position.
- Result: An element using the
moveAndColor
animation will start blue and in its original position, change to yellow and move 100px right in the middle, and then end up red and back in its original position.
2. Controlling Animation Speed: animation-timing-function
Once you've defined the steps of your animation with @keyframes
, you need to tell the browser how to transition between these steps. The animation-timing-function
property lets you control the speed curve, or the pace, of the animation.
/* Apply 'moveAndColor' to an element with class="my-element" */
.my-element {
animation-name: moveAndColor; /* Which @keyframes to use */
animation-duration: 4s; /* How long one cycle takes (4 seconds) */
/* Control the speed curve */
animation-timing-function: ease-in-out; /* Starts slow, speeds up, ends slow */
/* Other options to try: */
/* animation-timing-function: linear; */ /* Constant speed */
/* animation-timing-function: ease-in; */ /* Starts slow, speeds up */
/* animation-timing-function: steps(5, end); */ /* Jumps in 5 distinct steps */
}
.my-element { ... }
: This CSS rule targets any HTML element with the classmy-element
.animation-name: moveAndColor;
: This tells the element to use the animation defined by the@keyframes
rule namedmoveAndColor
.animation-duration: 4s;
: This sets the total time for one complete run of the animation to be 4 seconds.animation-timing-function: ease-in-out;
: This controls how the animation progresses over those 4 seconds.ease-in-out
is a common choice because it makes the animation start slowly, speed up in the middle, and then slow down again at the end, which often looks more natural.- The commented-out lines show other possible values for
animation-timing-function
:linear
: The animation proceeds at a constant speed from start to finish.ease-in
: The animation starts slowly and then speeds up.steps(5, end)
: Instead of a smooth transition, the animation jumps through 5 distinct steps, staying at each step for an equal amount of time. Theend
keyword means the change happens at the end of each step's duration.
- Result: The
.my-element
will perform themoveAndColor
animation over 4 seconds, starting and ending smoothly.
3. Animation vs. Transitions: Understanding the Difference
While both CSS animations and transitions allow you to create visual changes over time, they serve different primary purposes and have key distinctions:
Feature | CSS Transitions | CSS Animations |
---|---|---|
Trigger | Triggered by a change in CSS property value (e.g., :hover , JavaScript modification). |
Start automatically when the element loads or when triggered by JavaScript. |
Keyframes | Implicit; define the start and end states. | Explicit; define multiple intermediate states (@keyframes ). |
Complexity | Best for simple, one-step changes. | Ideal for complex sequences and multiple style changes. |
Control | Limited control during the transition. | Fine-grained control over each step and the overall timeline. |
Iteration | Typically run once. | Can be set to repeat (finite or infinite). |
Direction | Usually forward, can be reversed with JavaScript. | Offers more direction control (alternate, reverse, etc.). |
In essence:
- Transitions are excellent for creating smooth responses to user interactions or dynamic state changes. Think of a button changing color on hover or an element sliding into view when triggered.
- Animations are more powerful for creating standalone visual effects, repeating elements, or complex sequences that aren't directly tied to a single property change triggered by an action. Think of a loading spinner, a bouncing icon, or a more elaborate UI flourish.
Choosing between them depends on the specific effect you want to achieve and how it should be triggered and controlled.
4. The Animation Shorthand Property
Writing out all the individual animation-
properties can make your CSS a bit lengthy. CSS offers the animation
shorthand property, which lets you set multiple animation properties in a single line.
.my-element {
/* --- Longhand Version --- */
/*
animation-name: moveAndColor;
animation-duration: 4s;
animation-timing-function: ease-in-out;
animation-delay: 1s; // Wait 1s before starting
animation-iteration-count: infinite; // Repeat forever
animation-direction: alternate; // Play forwards, then backwards, repeat
animation-fill-mode: both; // Apply styles before/after animation
animation-play-state: running; // Animation plays by default
*/
/* --- Shorthand Version --- */
animation: moveAndColor 4s ease-in-out 1s infinite alternate both running;
}
/* Another example: Fade in and stay visible */
.another-element {
/* Applies 'fadeIn', takes 2s, 'ease-out' timing, stays faded in */
animation: fadeIn 2s ease-out forwards;
}
- Longhand: This section shows the individual animation properties for clarity.
- Shorthand (
animation: ...
): This combines the values of several animation properties into one declaration. While the order is somewhat flexible, it's generally recommended to haveanimation-name
andanimation-duration
early in the list.moveAndColor
: This is theanimation-name
, referring to our@keyframes
rule.4s
: This is theanimation-duration
, setting the animation cycle to 4 seconds.ease-in-out
: This is theanimation-timing-function
, controlling the speed curve.1s
: This is theanimation-delay
, meaning the animation will wait for 1 second after the element appears before starting.infinite
: This is theanimation-iteration-count
, telling the animation to repeat endlessly.alternate
: This is theanimation-direction
. It makes the animation play forwards on the first iteration, then backwards on the second, then forwards again, and so on.both
: This is theanimation-fill-mode
. It dictates that the styles from the first keyframe (0%
) should be applied during theanimation-delay
, and the styles from the last keyframe (100%
) should be maintained after the animation completes each iteration (in the alternating directions).running
: This is theanimation-play-state
, indicating that the animation should start playing as soon as the element loads.
.another-element
Example:forwards
: This is another value foranimation-fill-mode
. It ensures that after thefadeIn
animation completes (reachingopacity: 1
), the element keeps those final styles. Withoutforwards
, the element might revert to its originalopacity
after the animation finishes.
- Result: The shorthand provides a more compact way to define all the animation properties for an element. The
.another-element
will fade in over 2 seconds using an ease-out timing function (starts fast and slows down) and then remain fully visible.
5. Controlling Playback: Pausing on Hover
You can also control the state of an animation dynamically. A common and useful technique is to pause an animation when a user interacts with an element, such as hovering their mouse over it.
/* Select the element only when the mouse is hovering over it */
.interactive-element:hover {
/* Pause the animation */
animation-play-state: paused;
}
.interactive-element:hover
: This is a CSS selector that targets elements with the classinteractive-element
specifically when the user's mouse cursor is positioned over them. The:hover
part is a "pseudo-class" that represents this specific state of user interaction.animation-play-state: paused;
: This CSS property sets the current state of any running animation on the selected element topaused
. When the mouse cursor moves away from the element, the:hover
state ends, this style rule is no longer applied, and the animation will typically resume from where it was paused (assuming its default state isrunning
).- Result: Any animation that is applied to an element with the class
interactive-element
will stop playing as long as the user's mouse is hovering over it and will continue playing once the mouse moves away.
Project: Loading Spiral Screen
Ok, now let's build a simple Loading Screen project!
We will create a CSS-only loading screen with a soft gray spiral loader and "Ujjwalit" text below it. It will sit at the center of the page, suitable for clean websites or modern dashboards.
Code Implementation
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Minimal Loading Screen</title>
<style>
body {
margin: 0;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
background-color: #ffffff;
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
}
.loading-container {
display: flex;
flex-direction: column;
align-items: center;
}
.loader {
width: 40px;
height: 40px;
border: 3px solid #ccc;
border-top-color: #333;
border-radius: 50%;
animation: spin 1s linear infinite;
margin-bottom: 16px;
}
@keyframes spin {
0% { transform: rotate(0deg); }
100% { transform: rotate(360deg); }
}
.text {
font-size: 18px;
color: #333;
color: orange;
letter-spacing: 0.5px;
}
</style>
</head>
<body>
<div class="loading-container">
<div class="loader"></div>
<div class="text">Ujjwalit</div>
</div>
</body>
</html>
How the Animation Works
-
Centered Layout:
body
uses Flexbox to center the loader and text both vertically and horizontally.
-
Spiral Loader:
- A circular
div
is styled withborder-radius: 50%
. - It has a light gray border and one dark top border to create a rotating effect.
animation: spin 1s linear infinite
spins it continuously.
- A circular
- And that's all for animation in CSS. Try building your own elements and styling them with animations.
Want to keep learning?
Sign up on our platform or join our WhatsApp channel here to get more hands-on guides like this, delivered regularly.
See you in the next blog. Until then, keep practicing and happy learning!
4 Reactions
2 Bookmarks